- Apache Httpclient Ssl Example
- Apache Httpclient Tutorial
- Apache Httpclient Ssl
- Apache Httpclient Ssl Certificate Verification
- Apache Httpclient Timeout
In some contexts, it might very useful to disable the SSL checks when connecting to https
using Java. In my case, I was creating a temporary test server using Docker, where the proxy in front of my application was an Nginx with a self signed certificate.
Of course, when connecting with Java, this call is rejected because:
Apache HttpClient is very widely used for sending HTTP requests from java program itself. If you are using Maven, then you can add below dependencies and it will include all other required dependencies for using Apache HttpClient. Java, apache httpclient, ssl certificate, http, api, ssl, client-side certificate, security Published at DZone with permission of Simone Pezzano, DZone MVB. See the original article here. HttpClient instances can be created either using HttpClientBuilder or factory methods of the HttpClients utility class. This code snippet shows how to create HttpClient instance with default configuration. The instance will be configured to use a pool of connections with maximum two concurrent connections for the same route (host). Apache HttpClient - User Authentication - Using HttpClient, you can connect to a website which needed username and password. This chapter explains, how to execute a client request against a site that as.
- the certificate chain is invalid
- the host name cannot be trusted
But the truth is: in this very context, I do not care.
Google phone contacts. Disclaimer: of course such checks MUST be enabled against a production-like environment or external system.
Using Apache HttpClient 4.x, disabling SSL checks is actually quite easy:
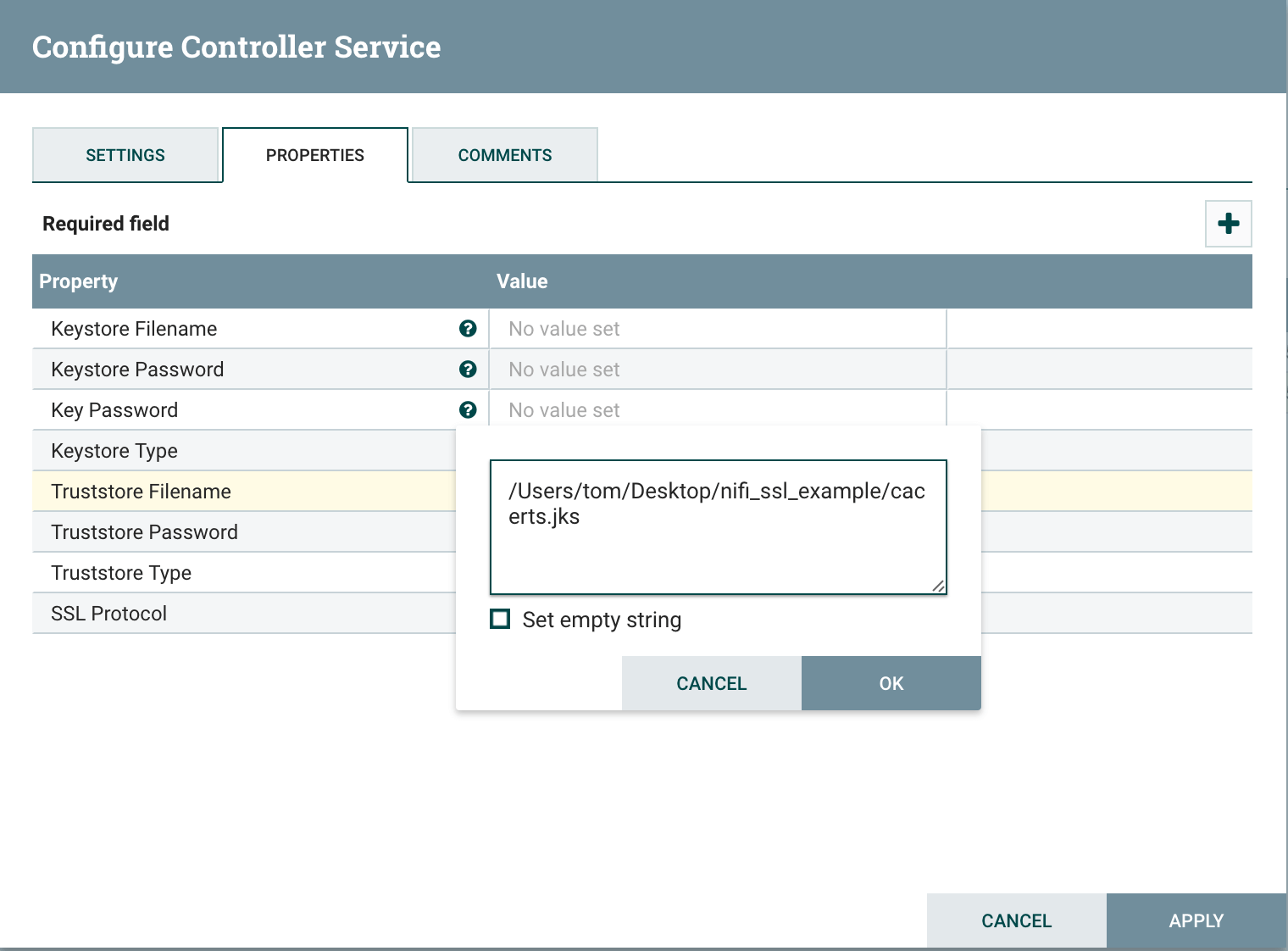
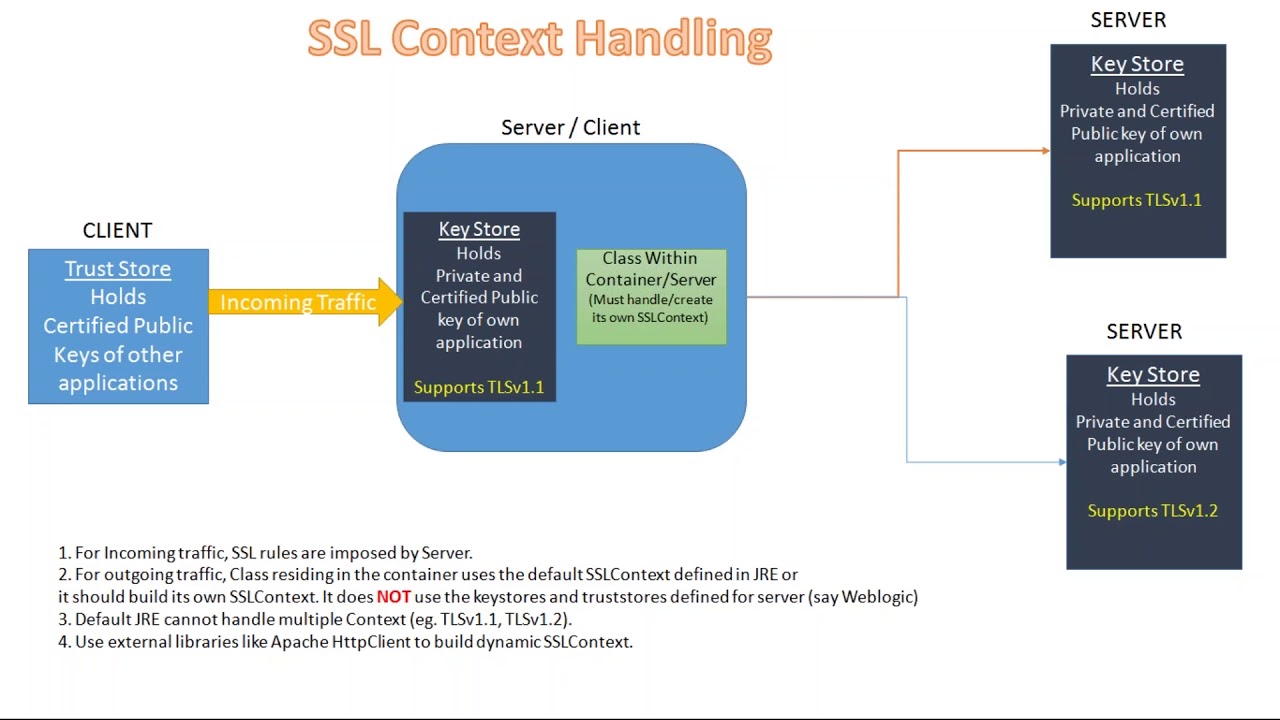
That's it. I fall back to the defaults when I do not want to disable the SSL checks.
- Apache HttpClient Tutorial
- Apache HttpClient Resources
- Selected Reading
Using the HttpClient library you can send a request or, login to a form by passing parameters.
Apache Httpclient Ssl Example
Follow the steps given below to login to a form. Soundgarden superunknown zip rar converter.
Step 1 - Create an HttpClient object
Apache Httpclient Tutorial
The createDefault() method of the HttpClients class returns an object of the class CloseableHttpClient, which is the base implementation of the HttpClient interface. Using this method, create an HttpClient object − Animal crossing new leaf mac download.
Step 2 - Create a RequestBuilder object
The class RequestBuilder is used to build request by adding parameters to it. If the request type is PUT or POST, it adds the parameters to the request as URL encoded entity
Create a RequestBuilder object (of type POST) using the post() method.
Step 3 - Set Uri and parameters to the RequestBuilder.
Set the URI and parameters to the RequestBuilder object using the setUri() and addParameter() methods of the RequestBuilder class.
Step 4 - Build the HttpUriRequest object
After setting the required parameters, build the HttpUriRequest object using the build() method.
Step 5 - Execute the request
The execute method of the CloseableHttpClient object accepts a HttpUriRequest (interface) object (i.e. HttpGet, HttpPost, HttpPut, HttpHead etc.) and returns a response object.
Execute the HttpUriRequest created in the previous steps by passing it to the execute()method.
Example
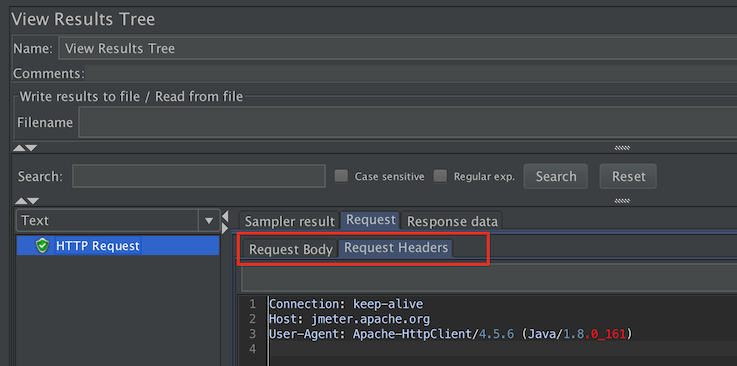
Following example demonstrates how to logon to a form by sending login credentials. Here, we have sent two parameters − username and password to a form and tried to print the message entity and status of the request.
Output
On executing, the above program generates the following output −
Form Login with Cookies
If your form stores cookies, instead of creating default CloseableHttpClient object.
Create a CookieStore object by instantiating the BasicCookieStore class.
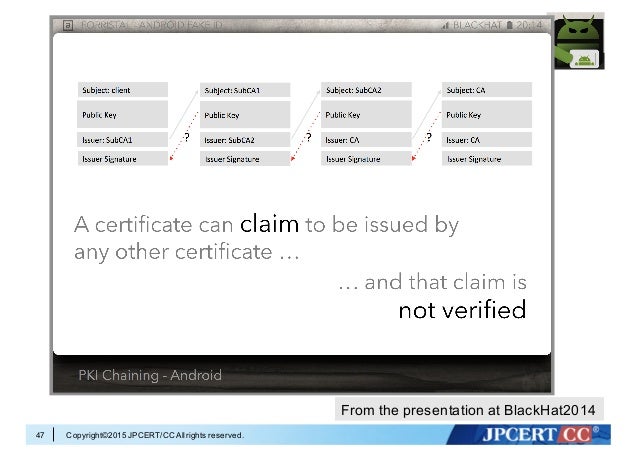
Apache Httpclient Ssl
Create a HttpClientBuilder using the custom() method of the HttpClients class.
Set the cookie store to the client builder using the setDefaultCookieStore() method.
Build the CloseableHttpClient object using the build() method.
Build the HttpUriRequest object as specified above by passing execute the request.
If the page stores cookies, the parameters you have passed will be added to the cookie store.
Apache Httpclient Ssl Certificate Verification
You can print the contents of the CookieStore object where you can see your parameters (along with the previous ones the page stored in case).
Apache Httpclient Timeout
To print the cookies, get all the cookies from the CookieStore object using the getCookies() method. This method returns a List object. Using Iterator, print the list objects contents as shown below −
